Insert or push_back to end of a std::vector?Does std::vector::insert reserve by definition?Concatenating two std::vectors“INSERT IGNORE” vs “INSERT … ON DUPLICATE KEY UPDATE”How to insert an item into an array at a specific index (JavaScript)?Efficiency of Java “Double Brace Initialization”?Why is “using namespace std;” considered bad practice?Improve INSERT-per-second performance of SQLite?push_back vs emplace_backImage Processing: Algorithm Improvement for 'Coca-Cola Can' RecognitionReplacing a 32-bit loop counter with 64-bit introduces crazy performance deviations
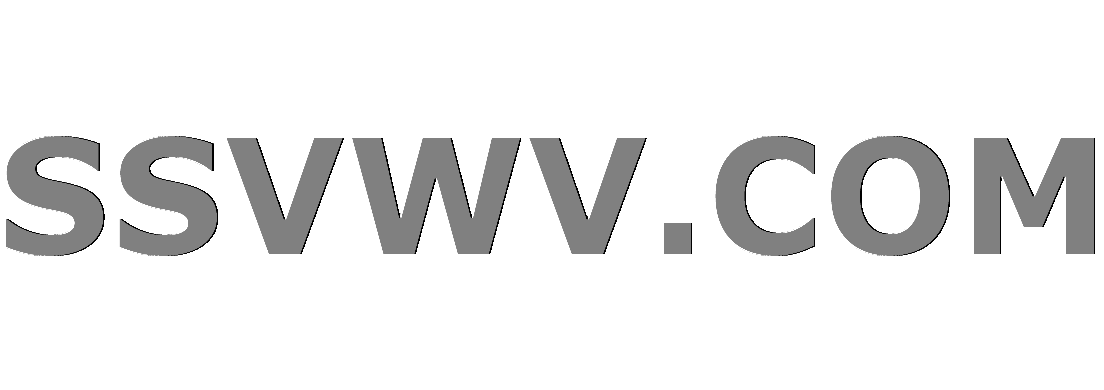
Multi tool use
How do I portray irrational anger in first person?
How could a self contained organic body propel itself in space
STM32 cannot reach individual registers and pins as PIC
Looking for a plural noun related to ‘fulcrum’ or ‘pivot’ that denotes multiple things as crucial to success
Which polygons can be turned inside out by a smooth deformation?
Why nature prefers simultaneous events?
Why does the weaker C–H bond have a higher wavenumber than the C=O bond?
Pen test results for web application include a file from a forbidden directory that is not even used or referenced
Why does Sauron not permit his followers to use his name?
How to export an email from Mail application?
Was the six engine Boeing-747 ever thought about?
Why is "I let him to sleep" incorrect (or is it)?
Why is there no Disney logo in MCU movies?
Why is there not a willingness from the world to step in between Pakistan and India?
Normalized Malbolge to Malbolge translator
Is this position a forced win for Black after move 14?
Why does glibc's strlen need to be so complicated to run quickly?
Count the number of triangles
What checks exist against overuse of presidential pardons in the USA?
Employing a contractor proving difficult
Shall I fix cracks on bathtub and how to fix them?
Why does this London Underground poster from 1924 have a Star of David atop a Christmas tree?
Is there a way to tell what frequency I need a PWM to be?
Are there any to-scale diagrams of the TRAPPIST-1 system?
Insert or push_back to end of a std::vector?
Does std::vector::insert reserve by definition?Concatenating two std::vectors“INSERT IGNORE” vs “INSERT … ON DUPLICATE KEY UPDATE”How to insert an item into an array at a specific index (JavaScript)?Efficiency of Java “Double Brace Initialization”?Why is “using namespace std;” considered bad practice?Improve INSERT-per-second performance of SQLite?push_back vs emplace_backImage Processing: Algorithm Improvement for 'Coca-Cola Can' RecognitionReplacing a 32-bit loop counter with 64-bit introduces crazy performance deviations
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
Is there any difference in performance between the two methods below to insert new elements to the end of a std::vector
:
Method 1
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
Method 2
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
Personally, I like method 2 because it is nice and concise and inserts all the new elements from an array in one go. But
- is there any difference in performance?
- After all, they do the same thing. Don't they?
Update
The reason why I am not initializing the vector with all the elements, to begin with, is that in my program I am adding the remaining elements based on a condition.
c++ performance c++11 stl insert
add a comment |
Is there any difference in performance between the two methods below to insert new elements to the end of a std::vector
:
Method 1
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
Method 2
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
Personally, I like method 2 because it is nice and concise and inserts all the new elements from an array in one go. But
- is there any difference in performance?
- After all, they do the same thing. Don't they?
Update
The reason why I am not initializing the vector with all the elements, to begin with, is that in my program I am adding the remaining elements based on a condition.
c++ performance c++11 stl insert
7
There is also another option:std::vector<int> vec 1,2,3,4,5 ;
– JeJo
Aug 16 at 8:34
10
Create a small test program where you do each a million times. Build with optimizations enabled, and test and measure.
– Some programmer dude
Aug 16 at 8:34
1
Oh and while the end result (a vector with five elements) the two methods do different things. The most important difference is the allocation and reallocation of the data needed for the elements in the vector. The first variant could lead to four reallocations, and with a possibly different capacity and size, while the second variant there's probably only a single reallocation and maybe the same capacity and size.
– Some programmer dude
Aug 16 at 8:38
1
Personally I like method 2 because it's nice and concise and inserts all the new elements from an array in one go. That's the important reason, performance is secondary.
– john
Aug 16 at 8:43
add a comment |
Is there any difference in performance between the two methods below to insert new elements to the end of a std::vector
:
Method 1
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
Method 2
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
Personally, I like method 2 because it is nice and concise and inserts all the new elements from an array in one go. But
- is there any difference in performance?
- After all, they do the same thing. Don't they?
Update
The reason why I am not initializing the vector with all the elements, to begin with, is that in my program I am adding the remaining elements based on a condition.
c++ performance c++11 stl insert
Is there any difference in performance between the two methods below to insert new elements to the end of a std::vector
:
Method 1
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
Method 2
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
Personally, I like method 2 because it is nice and concise and inserts all the new elements from an array in one go. But
- is there any difference in performance?
- After all, they do the same thing. Don't they?
Update
The reason why I am not initializing the vector with all the elements, to begin with, is that in my program I am adding the remaining elements based on a condition.
c++ performance c++11 stl insert
c++ performance c++11 stl insert
edited Aug 20 at 7:37


JeJo
9,6863 gold badges15 silver badges45 bronze badges
9,6863 gold badges15 silver badges45 bronze badges
asked Aug 16 at 8:32


jacobijacobi
4682 silver badges12 bronze badges
4682 silver badges12 bronze badges
7
There is also another option:std::vector<int> vec 1,2,3,4,5 ;
– JeJo
Aug 16 at 8:34
10
Create a small test program where you do each a million times. Build with optimizations enabled, and test and measure.
– Some programmer dude
Aug 16 at 8:34
1
Oh and while the end result (a vector with five elements) the two methods do different things. The most important difference is the allocation and reallocation of the data needed for the elements in the vector. The first variant could lead to four reallocations, and with a possibly different capacity and size, while the second variant there's probably only a single reallocation and maybe the same capacity and size.
– Some programmer dude
Aug 16 at 8:38
1
Personally I like method 2 because it's nice and concise and inserts all the new elements from an array in one go. That's the important reason, performance is secondary.
– john
Aug 16 at 8:43
add a comment |
7
There is also another option:std::vector<int> vec 1,2,3,4,5 ;
– JeJo
Aug 16 at 8:34
10
Create a small test program where you do each a million times. Build with optimizations enabled, and test and measure.
– Some programmer dude
Aug 16 at 8:34
1
Oh and while the end result (a vector with five elements) the two methods do different things. The most important difference is the allocation and reallocation of the data needed for the elements in the vector. The first variant could lead to four reallocations, and with a possibly different capacity and size, while the second variant there's probably only a single reallocation and maybe the same capacity and size.
– Some programmer dude
Aug 16 at 8:38
1
Personally I like method 2 because it's nice and concise and inserts all the new elements from an array in one go. That's the important reason, performance is secondary.
– john
Aug 16 at 8:43
7
7
There is also another option:
std::vector<int> vec 1,2,3,4,5 ;
– JeJo
Aug 16 at 8:34
There is also another option:
std::vector<int> vec 1,2,3,4,5 ;
– JeJo
Aug 16 at 8:34
10
10
Create a small test program where you do each a million times. Build with optimizations enabled, and test and measure.
– Some programmer dude
Aug 16 at 8:34
Create a small test program where you do each a million times. Build with optimizations enabled, and test and measure.
– Some programmer dude
Aug 16 at 8:34
1
1
Oh and while the end result (a vector with five elements) the two methods do different things. The most important difference is the allocation and reallocation of the data needed for the elements in the vector. The first variant could lead to four reallocations, and with a possibly different capacity and size, while the second variant there's probably only a single reallocation and maybe the same capacity and size.
– Some programmer dude
Aug 16 at 8:38
Oh and while the end result (a vector with five elements) the two methods do different things. The most important difference is the allocation and reallocation of the data needed for the elements in the vector. The first variant could lead to four reallocations, and with a possibly different capacity and size, while the second variant there's probably only a single reallocation and maybe the same capacity and size.
– Some programmer dude
Aug 16 at 8:38
1
1
Personally I like method 2 because it's nice and concise and inserts all the new elements from an array in one go. That's the important reason, performance is secondary.
– john
Aug 16 at 8:43
Personally I like method 2 because it's nice and concise and inserts all the new elements from an array in one go. That's the important reason, performance is secondary.
– john
Aug 16 at 8:43
add a comment |
4 Answers
4
active
oldest
votes
There may be a difference between the two approaches if the vector needs to reallocate.
Your second method, calling the insert()
member function once with an iterator range:
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
would be able to provide the optimisation of allocating all the memory needed for the insertion of the elements in one blow since insert()
is getting random access iterators, i.e., it takes constant time to know the size of the range, so the whole memory allocation can be done before copying the elements, and no reallocations during the call would follow.
Your first method, individual calls to the push_back()
member function, may trigger several reallocations, depending on the number of elements to insert and the memory initially reserved for the vector.
Note that the optimisation explained above may not be available for forward or bidirectional iterators since it would take linear time in the size of the range to know the number of elements to be inserted. However, the time needed for multiple memory allocations likely dwarfs the time needed to calculate the length of the range for these cases, so probably they still implement this optimisation. For input iterators, this optimisation is not even possible since they are single-pass iterators.
add a comment |
After all, they do the same thing. Don't they?
No. They are different. The first method using std::vector::push_back
will undergo several reallocations compared to std::vector::insert
.
The insert
will internally allocate memory, according to the current std::vector::capacity
before copying the range. See the following discussion for more:
Does std::vector::insert reserve by definition?
But is there any difference in performance?
Due to the reason explained above, the second method would show slight performance improvement. For instance, see the quick benck-mark below, using http://quick-bench.com:
See online bench-mark
Or write a test program to measure the performance(as @Some programmer dude mentioned in the comments). Following is a sample test program:
#include <iostream>
#include <chrono>
#include <algorithm>
#include <vector>
using namespace std::chrono;
class Timer final
private:
time_point<high_resolution_clock> _startTime;
public:
Timer() noexcept
: _startTime high_resolution_clock::now()
~Timer() noexcept Stop();
void Stop() noexcept
const auto endTime = high_resolution_clock::now();
const auto start = time_point_cast<microseconds>(_startTime).time_since_epoch();
const auto end = time_point_cast<microseconds>(endTime).time_since_epoch();
const auto durationTaken = end - start;
const auto duration_ms = durationTaken * 0.001;
std::cout << durationTaken.count() << "us (" << duration_ms.count() << "ms)n";
;
// Method 1: push_back
void push_back()
std::cout << "push_backing: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
// Method 2: insert_range
void insert_range()
std::cout << "range-inserting: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::cbegin(arr), std::cend(arr));
int main()
push_back();
insert_range();
return 0;
release building with my system(MSVS2019:/Ox /std:c++17, AMD Ryzen 7 2700x(8-core, 3.70 Ghz), x64 Windows 10)
// Build - 1
push_backing: 285199us (285.199ms)
range-inserting: 103388us (103.388ms)
// Build - 2
push_backing: 280378us (280.378ms)
range-inserting: 104032us (104.032ms)
// Build - 3
push_backing: 281818us (281.818ms)
range-inserting: 102803us (102.803ms)
Which shows for the given scenario, std::vector::insert
ing is about 2.7
times faster than std::vector::push_back
.
See what other compilers(clang 8.0 and gcc 9.2) wants to say, according to their implementations: https://godbolt.org/z/DQrq51
1
Wow! The graphs show that insert is much better in performance in this case.
– jacobi
Aug 16 at 9:07
3
@jacobi Yeap. Also note that thestd::vector:::reserve
will bring the performance at the same level. See here Therefore reserve the memory, if you know the size beforehand, and avoid unwanted reallocations.
– JeJo
Aug 16 at 9:09
@JeJo: Note also that, from your link: "When inserting a range, the range version ofinsert()
is generally preferable as it preserves the correct capacity growth behavior, unlikereserve()
followed by a series ofpush_back()
s." In other words,reserve()
will either cause a realloc or do nothing, and if it causes a realloc, you will probably end up with a full or nearly full vector after doing thepush_back()
calls. That is wasteful since it means you'll be doing another realloc in the near future.
– Kevin
Aug 16 at 17:51
@mirabilos the purpose of the website is to give a comparison between the code snippets, rather providing an accurate x-y axis measures. Incase of accurate benchmarking, one should write a test program and has to measure for certain times. For instance, further interests, following is a test-program that I tried: godbolt.org/z/DQrq51
– JeJo
Aug 16 at 21:00
@JeJo ah, thanks. I didn’t follow the link because what’s supplied on SO “ought to be enough” and most of the external things don’t work on my browser anyway. The changed image will do, thanks! (So, smaller bar = better.)
– mirabilos
Aug 17 at 14:20
add a comment |
push_back
inserts a single element, hence in the worst case you may encounter multiple reallocations.
For the sake of the example, consider the case where the initial capacity is 2 and increases by a factor of 2 on each reallocation. Then
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3); // need to reallocate, capacity is 4
vec.push_back(4);
vec.push_back(5); // need to reallocate, capacity is 8
You can of course prevent unnecessary reallocations by calling
vec.reserve(num_elements_to_push);
Though, if you anyhow insert from an array, the more idomatic way is to use insert
.
add a comment |
The major contributing factor is going to be the re-allocations. vector
has to make space for new elements.
Consider these 3 sinppets.
//pushback
std::vector<int> vec = 1;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
//insert
std::vector<int> vec = 1;
int arr[] = 2,3,4,5;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
//cosntruct
std::vector<int> vec = 1,2,3,4,5;
To confirm the reallocations coming into picture, after adding a vec.reserve(5)
in pushback and insert versions, we get the below results.
Interesting how adding reserve brings down the time for push_back and insert to similar levels.
– jacobi
Aug 16 at 9:27
1
Why would one want to addreserve()
to theinsert()
version? I don't get the same performance hit as you when doing it though: quick-bench.com/4ioxDEyzxMl37C7eHfNICKk0Tpc
– Ted Lyngmo
Aug 16 at 9:32
@TedLyngmo I did with clang. Don't know whyclang
's insert is slower.
– Gaurav Sehgal
Aug 16 at 10:57
1
Wow, yeah, that was a surprise! ... and usingclang++
+libc++(LLVM)
made it even worse: quick-bench.com/jfcVMGkhgFRI33-2PMPR74cM1G0
– Ted Lyngmo
Aug 16 at 11:44
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f57521335%2finsert-or-push-back-to-end-of-a-stdvector%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
There may be a difference between the two approaches if the vector needs to reallocate.
Your second method, calling the insert()
member function once with an iterator range:
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
would be able to provide the optimisation of allocating all the memory needed for the insertion of the elements in one blow since insert()
is getting random access iterators, i.e., it takes constant time to know the size of the range, so the whole memory allocation can be done before copying the elements, and no reallocations during the call would follow.
Your first method, individual calls to the push_back()
member function, may trigger several reallocations, depending on the number of elements to insert and the memory initially reserved for the vector.
Note that the optimisation explained above may not be available for forward or bidirectional iterators since it would take linear time in the size of the range to know the number of elements to be inserted. However, the time needed for multiple memory allocations likely dwarfs the time needed to calculate the length of the range for these cases, so probably they still implement this optimisation. For input iterators, this optimisation is not even possible since they are single-pass iterators.
add a comment |
There may be a difference between the two approaches if the vector needs to reallocate.
Your second method, calling the insert()
member function once with an iterator range:
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
would be able to provide the optimisation of allocating all the memory needed for the insertion of the elements in one blow since insert()
is getting random access iterators, i.e., it takes constant time to know the size of the range, so the whole memory allocation can be done before copying the elements, and no reallocations during the call would follow.
Your first method, individual calls to the push_back()
member function, may trigger several reallocations, depending on the number of elements to insert and the memory initially reserved for the vector.
Note that the optimisation explained above may not be available for forward or bidirectional iterators since it would take linear time in the size of the range to know the number of elements to be inserted. However, the time needed for multiple memory allocations likely dwarfs the time needed to calculate the length of the range for these cases, so probably they still implement this optimisation. For input iterators, this optimisation is not even possible since they are single-pass iterators.
add a comment |
There may be a difference between the two approaches if the vector needs to reallocate.
Your second method, calling the insert()
member function once with an iterator range:
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
would be able to provide the optimisation of allocating all the memory needed for the insertion of the elements in one blow since insert()
is getting random access iterators, i.e., it takes constant time to know the size of the range, so the whole memory allocation can be done before copying the elements, and no reallocations during the call would follow.
Your first method, individual calls to the push_back()
member function, may trigger several reallocations, depending on the number of elements to insert and the memory initially reserved for the vector.
Note that the optimisation explained above may not be available for forward or bidirectional iterators since it would take linear time in the size of the range to know the number of elements to be inserted. However, the time needed for multiple memory allocations likely dwarfs the time needed to calculate the length of the range for these cases, so probably they still implement this optimisation. For input iterators, this optimisation is not even possible since they are single-pass iterators.
There may be a difference between the two approaches if the vector needs to reallocate.
Your second method, calling the insert()
member function once with an iterator range:
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
would be able to provide the optimisation of allocating all the memory needed for the insertion of the elements in one blow since insert()
is getting random access iterators, i.e., it takes constant time to know the size of the range, so the whole memory allocation can be done before copying the elements, and no reallocations during the call would follow.
Your first method, individual calls to the push_back()
member function, may trigger several reallocations, depending on the number of elements to insert and the memory initially reserved for the vector.
Note that the optimisation explained above may not be available for forward or bidirectional iterators since it would take linear time in the size of the range to know the number of elements to be inserted. However, the time needed for multiple memory allocations likely dwarfs the time needed to calculate the length of the range for these cases, so probably they still implement this optimisation. For input iterators, this optimisation is not even possible since they are single-pass iterators.
edited Aug 16 at 8:59
answered Aug 16 at 8:42


El ProfesorEl Profesor
11.8k3 gold badges29 silver badges43 bronze badges
11.8k3 gold badges29 silver badges43 bronze badges
add a comment |
add a comment |
After all, they do the same thing. Don't they?
No. They are different. The first method using std::vector::push_back
will undergo several reallocations compared to std::vector::insert
.
The insert
will internally allocate memory, according to the current std::vector::capacity
before copying the range. See the following discussion for more:
Does std::vector::insert reserve by definition?
But is there any difference in performance?
Due to the reason explained above, the second method would show slight performance improvement. For instance, see the quick benck-mark below, using http://quick-bench.com:
See online bench-mark
Or write a test program to measure the performance(as @Some programmer dude mentioned in the comments). Following is a sample test program:
#include <iostream>
#include <chrono>
#include <algorithm>
#include <vector>
using namespace std::chrono;
class Timer final
private:
time_point<high_resolution_clock> _startTime;
public:
Timer() noexcept
: _startTime high_resolution_clock::now()
~Timer() noexcept Stop();
void Stop() noexcept
const auto endTime = high_resolution_clock::now();
const auto start = time_point_cast<microseconds>(_startTime).time_since_epoch();
const auto end = time_point_cast<microseconds>(endTime).time_since_epoch();
const auto durationTaken = end - start;
const auto duration_ms = durationTaken * 0.001;
std::cout << durationTaken.count() << "us (" << duration_ms.count() << "ms)n";
;
// Method 1: push_back
void push_back()
std::cout << "push_backing: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
// Method 2: insert_range
void insert_range()
std::cout << "range-inserting: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::cbegin(arr), std::cend(arr));
int main()
push_back();
insert_range();
return 0;
release building with my system(MSVS2019:/Ox /std:c++17, AMD Ryzen 7 2700x(8-core, 3.70 Ghz), x64 Windows 10)
// Build - 1
push_backing: 285199us (285.199ms)
range-inserting: 103388us (103.388ms)
// Build - 2
push_backing: 280378us (280.378ms)
range-inserting: 104032us (104.032ms)
// Build - 3
push_backing: 281818us (281.818ms)
range-inserting: 102803us (102.803ms)
Which shows for the given scenario, std::vector::insert
ing is about 2.7
times faster than std::vector::push_back
.
See what other compilers(clang 8.0 and gcc 9.2) wants to say, according to their implementations: https://godbolt.org/z/DQrq51
1
Wow! The graphs show that insert is much better in performance in this case.
– jacobi
Aug 16 at 9:07
3
@jacobi Yeap. Also note that thestd::vector:::reserve
will bring the performance at the same level. See here Therefore reserve the memory, if you know the size beforehand, and avoid unwanted reallocations.
– JeJo
Aug 16 at 9:09
@JeJo: Note also that, from your link: "When inserting a range, the range version ofinsert()
is generally preferable as it preserves the correct capacity growth behavior, unlikereserve()
followed by a series ofpush_back()
s." In other words,reserve()
will either cause a realloc or do nothing, and if it causes a realloc, you will probably end up with a full or nearly full vector after doing thepush_back()
calls. That is wasteful since it means you'll be doing another realloc in the near future.
– Kevin
Aug 16 at 17:51
@mirabilos the purpose of the website is to give a comparison between the code snippets, rather providing an accurate x-y axis measures. Incase of accurate benchmarking, one should write a test program and has to measure for certain times. For instance, further interests, following is a test-program that I tried: godbolt.org/z/DQrq51
– JeJo
Aug 16 at 21:00
@JeJo ah, thanks. I didn’t follow the link because what’s supplied on SO “ought to be enough” and most of the external things don’t work on my browser anyway. The changed image will do, thanks! (So, smaller bar = better.)
– mirabilos
Aug 17 at 14:20
add a comment |
After all, they do the same thing. Don't they?
No. They are different. The first method using std::vector::push_back
will undergo several reallocations compared to std::vector::insert
.
The insert
will internally allocate memory, according to the current std::vector::capacity
before copying the range. See the following discussion for more:
Does std::vector::insert reserve by definition?
But is there any difference in performance?
Due to the reason explained above, the second method would show slight performance improvement. For instance, see the quick benck-mark below, using http://quick-bench.com:
See online bench-mark
Or write a test program to measure the performance(as @Some programmer dude mentioned in the comments). Following is a sample test program:
#include <iostream>
#include <chrono>
#include <algorithm>
#include <vector>
using namespace std::chrono;
class Timer final
private:
time_point<high_resolution_clock> _startTime;
public:
Timer() noexcept
: _startTime high_resolution_clock::now()
~Timer() noexcept Stop();
void Stop() noexcept
const auto endTime = high_resolution_clock::now();
const auto start = time_point_cast<microseconds>(_startTime).time_since_epoch();
const auto end = time_point_cast<microseconds>(endTime).time_since_epoch();
const auto durationTaken = end - start;
const auto duration_ms = durationTaken * 0.001;
std::cout << durationTaken.count() << "us (" << duration_ms.count() << "ms)n";
;
// Method 1: push_back
void push_back()
std::cout << "push_backing: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
// Method 2: insert_range
void insert_range()
std::cout << "range-inserting: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::cbegin(arr), std::cend(arr));
int main()
push_back();
insert_range();
return 0;
release building with my system(MSVS2019:/Ox /std:c++17, AMD Ryzen 7 2700x(8-core, 3.70 Ghz), x64 Windows 10)
// Build - 1
push_backing: 285199us (285.199ms)
range-inserting: 103388us (103.388ms)
// Build - 2
push_backing: 280378us (280.378ms)
range-inserting: 104032us (104.032ms)
// Build - 3
push_backing: 281818us (281.818ms)
range-inserting: 102803us (102.803ms)
Which shows for the given scenario, std::vector::insert
ing is about 2.7
times faster than std::vector::push_back
.
See what other compilers(clang 8.0 and gcc 9.2) wants to say, according to their implementations: https://godbolt.org/z/DQrq51
1
Wow! The graphs show that insert is much better in performance in this case.
– jacobi
Aug 16 at 9:07
3
@jacobi Yeap. Also note that thestd::vector:::reserve
will bring the performance at the same level. See here Therefore reserve the memory, if you know the size beforehand, and avoid unwanted reallocations.
– JeJo
Aug 16 at 9:09
@JeJo: Note also that, from your link: "When inserting a range, the range version ofinsert()
is generally preferable as it preserves the correct capacity growth behavior, unlikereserve()
followed by a series ofpush_back()
s." In other words,reserve()
will either cause a realloc or do nothing, and if it causes a realloc, you will probably end up with a full or nearly full vector after doing thepush_back()
calls. That is wasteful since it means you'll be doing another realloc in the near future.
– Kevin
Aug 16 at 17:51
@mirabilos the purpose of the website is to give a comparison between the code snippets, rather providing an accurate x-y axis measures. Incase of accurate benchmarking, one should write a test program and has to measure for certain times. For instance, further interests, following is a test-program that I tried: godbolt.org/z/DQrq51
– JeJo
Aug 16 at 21:00
@JeJo ah, thanks. I didn’t follow the link because what’s supplied on SO “ought to be enough” and most of the external things don’t work on my browser anyway. The changed image will do, thanks! (So, smaller bar = better.)
– mirabilos
Aug 17 at 14:20
add a comment |
After all, they do the same thing. Don't they?
No. They are different. The first method using std::vector::push_back
will undergo several reallocations compared to std::vector::insert
.
The insert
will internally allocate memory, according to the current std::vector::capacity
before copying the range. See the following discussion for more:
Does std::vector::insert reserve by definition?
But is there any difference in performance?
Due to the reason explained above, the second method would show slight performance improvement. For instance, see the quick benck-mark below, using http://quick-bench.com:
See online bench-mark
Or write a test program to measure the performance(as @Some programmer dude mentioned in the comments). Following is a sample test program:
#include <iostream>
#include <chrono>
#include <algorithm>
#include <vector>
using namespace std::chrono;
class Timer final
private:
time_point<high_resolution_clock> _startTime;
public:
Timer() noexcept
: _startTime high_resolution_clock::now()
~Timer() noexcept Stop();
void Stop() noexcept
const auto endTime = high_resolution_clock::now();
const auto start = time_point_cast<microseconds>(_startTime).time_since_epoch();
const auto end = time_point_cast<microseconds>(endTime).time_since_epoch();
const auto durationTaken = end - start;
const auto duration_ms = durationTaken * 0.001;
std::cout << durationTaken.count() << "us (" << duration_ms.count() << "ms)n";
;
// Method 1: push_back
void push_back()
std::cout << "push_backing: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
// Method 2: insert_range
void insert_range()
std::cout << "range-inserting: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::cbegin(arr), std::cend(arr));
int main()
push_back();
insert_range();
return 0;
release building with my system(MSVS2019:/Ox /std:c++17, AMD Ryzen 7 2700x(8-core, 3.70 Ghz), x64 Windows 10)
// Build - 1
push_backing: 285199us (285.199ms)
range-inserting: 103388us (103.388ms)
// Build - 2
push_backing: 280378us (280.378ms)
range-inserting: 104032us (104.032ms)
// Build - 3
push_backing: 281818us (281.818ms)
range-inserting: 102803us (102.803ms)
Which shows for the given scenario, std::vector::insert
ing is about 2.7
times faster than std::vector::push_back
.
See what other compilers(clang 8.0 and gcc 9.2) wants to say, according to their implementations: https://godbolt.org/z/DQrq51
After all, they do the same thing. Don't they?
No. They are different. The first method using std::vector::push_back
will undergo several reallocations compared to std::vector::insert
.
The insert
will internally allocate memory, according to the current std::vector::capacity
before copying the range. See the following discussion for more:
Does std::vector::insert reserve by definition?
But is there any difference in performance?
Due to the reason explained above, the second method would show slight performance improvement. For instance, see the quick benck-mark below, using http://quick-bench.com:
See online bench-mark
Or write a test program to measure the performance(as @Some programmer dude mentioned in the comments). Following is a sample test program:
#include <iostream>
#include <chrono>
#include <algorithm>
#include <vector>
using namespace std::chrono;
class Timer final
private:
time_point<high_resolution_clock> _startTime;
public:
Timer() noexcept
: _startTime high_resolution_clock::now()
~Timer() noexcept Stop();
void Stop() noexcept
const auto endTime = high_resolution_clock::now();
const auto start = time_point_cast<microseconds>(_startTime).time_since_epoch();
const auto end = time_point_cast<microseconds>(endTime).time_since_epoch();
const auto durationTaken = end - start;
const auto duration_ms = durationTaken * 0.001;
std::cout << durationTaken.count() << "us (" << duration_ms.count() << "ms)n";
;
// Method 1: push_back
void push_back()
std::cout << "push_backing: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
// Method 2: insert_range
void insert_range()
std::cout << "range-inserting: ";
Timer time;
for (auto i 0ULL ; i < 1000'000; ++i)
std::vector<int> vec = 1 ;
int arr[] = 2,3,4,5 ;
vec.insert(std::end(vec), std::cbegin(arr), std::cend(arr));
int main()
push_back();
insert_range();
return 0;
release building with my system(MSVS2019:/Ox /std:c++17, AMD Ryzen 7 2700x(8-core, 3.70 Ghz), x64 Windows 10)
// Build - 1
push_backing: 285199us (285.199ms)
range-inserting: 103388us (103.388ms)
// Build - 2
push_backing: 280378us (280.378ms)
range-inserting: 104032us (104.032ms)
// Build - 3
push_backing: 281818us (281.818ms)
range-inserting: 102803us (102.803ms)
Which shows for the given scenario, std::vector::insert
ing is about 2.7
times faster than std::vector::push_back
.
See what other compilers(clang 8.0 and gcc 9.2) wants to say, according to their implementations: https://godbolt.org/z/DQrq51
edited Aug 17 at 7:59
answered Aug 16 at 8:55


JeJoJeJo
9,6863 gold badges15 silver badges45 bronze badges
9,6863 gold badges15 silver badges45 bronze badges
1
Wow! The graphs show that insert is much better in performance in this case.
– jacobi
Aug 16 at 9:07
3
@jacobi Yeap. Also note that thestd::vector:::reserve
will bring the performance at the same level. See here Therefore reserve the memory, if you know the size beforehand, and avoid unwanted reallocations.
– JeJo
Aug 16 at 9:09
@JeJo: Note also that, from your link: "When inserting a range, the range version ofinsert()
is generally preferable as it preserves the correct capacity growth behavior, unlikereserve()
followed by a series ofpush_back()
s." In other words,reserve()
will either cause a realloc or do nothing, and if it causes a realloc, you will probably end up with a full or nearly full vector after doing thepush_back()
calls. That is wasteful since it means you'll be doing another realloc in the near future.
– Kevin
Aug 16 at 17:51
@mirabilos the purpose of the website is to give a comparison between the code snippets, rather providing an accurate x-y axis measures. Incase of accurate benchmarking, one should write a test program and has to measure for certain times. For instance, further interests, following is a test-program that I tried: godbolt.org/z/DQrq51
– JeJo
Aug 16 at 21:00
@JeJo ah, thanks. I didn’t follow the link because what’s supplied on SO “ought to be enough” and most of the external things don’t work on my browser anyway. The changed image will do, thanks! (So, smaller bar = better.)
– mirabilos
Aug 17 at 14:20
add a comment |
1
Wow! The graphs show that insert is much better in performance in this case.
– jacobi
Aug 16 at 9:07
3
@jacobi Yeap. Also note that thestd::vector:::reserve
will bring the performance at the same level. See here Therefore reserve the memory, if you know the size beforehand, and avoid unwanted reallocations.
– JeJo
Aug 16 at 9:09
@JeJo: Note also that, from your link: "When inserting a range, the range version ofinsert()
is generally preferable as it preserves the correct capacity growth behavior, unlikereserve()
followed by a series ofpush_back()
s." In other words,reserve()
will either cause a realloc or do nothing, and if it causes a realloc, you will probably end up with a full or nearly full vector after doing thepush_back()
calls. That is wasteful since it means you'll be doing another realloc in the near future.
– Kevin
Aug 16 at 17:51
@mirabilos the purpose of the website is to give a comparison between the code snippets, rather providing an accurate x-y axis measures. Incase of accurate benchmarking, one should write a test program and has to measure for certain times. For instance, further interests, following is a test-program that I tried: godbolt.org/z/DQrq51
– JeJo
Aug 16 at 21:00
@JeJo ah, thanks. I didn’t follow the link because what’s supplied on SO “ought to be enough” and most of the external things don’t work on my browser anyway. The changed image will do, thanks! (So, smaller bar = better.)
– mirabilos
Aug 17 at 14:20
1
1
Wow! The graphs show that insert is much better in performance in this case.
– jacobi
Aug 16 at 9:07
Wow! The graphs show that insert is much better in performance in this case.
– jacobi
Aug 16 at 9:07
3
3
@jacobi Yeap. Also note that the
std::vector:::reserve
will bring the performance at the same level. See here Therefore reserve the memory, if you know the size beforehand, and avoid unwanted reallocations.– JeJo
Aug 16 at 9:09
@jacobi Yeap. Also note that the
std::vector:::reserve
will bring the performance at the same level. See here Therefore reserve the memory, if you know the size beforehand, and avoid unwanted reallocations.– JeJo
Aug 16 at 9:09
@JeJo: Note also that, from your link: "When inserting a range, the range version of
insert()
is generally preferable as it preserves the correct capacity growth behavior, unlike reserve()
followed by a series of push_back()
s." In other words, reserve()
will either cause a realloc or do nothing, and if it causes a realloc, you will probably end up with a full or nearly full vector after doing the push_back()
calls. That is wasteful since it means you'll be doing another realloc in the near future.– Kevin
Aug 16 at 17:51
@JeJo: Note also that, from your link: "When inserting a range, the range version of
insert()
is generally preferable as it preserves the correct capacity growth behavior, unlike reserve()
followed by a series of push_back()
s." In other words, reserve()
will either cause a realloc or do nothing, and if it causes a realloc, you will probably end up with a full or nearly full vector after doing the push_back()
calls. That is wasteful since it means you'll be doing another realloc in the near future.– Kevin
Aug 16 at 17:51
@mirabilos the purpose of the website is to give a comparison between the code snippets, rather providing an accurate x-y axis measures. Incase of accurate benchmarking, one should write a test program and has to measure for certain times. For instance, further interests, following is a test-program that I tried: godbolt.org/z/DQrq51
– JeJo
Aug 16 at 21:00
@mirabilos the purpose of the website is to give a comparison between the code snippets, rather providing an accurate x-y axis measures. Incase of accurate benchmarking, one should write a test program and has to measure for certain times. For instance, further interests, following is a test-program that I tried: godbolt.org/z/DQrq51
– JeJo
Aug 16 at 21:00
@JeJo ah, thanks. I didn’t follow the link because what’s supplied on SO “ought to be enough” and most of the external things don’t work on my browser anyway. The changed image will do, thanks! (So, smaller bar = better.)
– mirabilos
Aug 17 at 14:20
@JeJo ah, thanks. I didn’t follow the link because what’s supplied on SO “ought to be enough” and most of the external things don’t work on my browser anyway. The changed image will do, thanks! (So, smaller bar = better.)
– mirabilos
Aug 17 at 14:20
add a comment |
push_back
inserts a single element, hence in the worst case you may encounter multiple reallocations.
For the sake of the example, consider the case where the initial capacity is 2 and increases by a factor of 2 on each reallocation. Then
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3); // need to reallocate, capacity is 4
vec.push_back(4);
vec.push_back(5); // need to reallocate, capacity is 8
You can of course prevent unnecessary reallocations by calling
vec.reserve(num_elements_to_push);
Though, if you anyhow insert from an array, the more idomatic way is to use insert
.
add a comment |
push_back
inserts a single element, hence in the worst case you may encounter multiple reallocations.
For the sake of the example, consider the case where the initial capacity is 2 and increases by a factor of 2 on each reallocation. Then
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3); // need to reallocate, capacity is 4
vec.push_back(4);
vec.push_back(5); // need to reallocate, capacity is 8
You can of course prevent unnecessary reallocations by calling
vec.reserve(num_elements_to_push);
Though, if you anyhow insert from an array, the more idomatic way is to use insert
.
add a comment |
push_back
inserts a single element, hence in the worst case you may encounter multiple reallocations.
For the sake of the example, consider the case where the initial capacity is 2 and increases by a factor of 2 on each reallocation. Then
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3); // need to reallocate, capacity is 4
vec.push_back(4);
vec.push_back(5); // need to reallocate, capacity is 8
You can of course prevent unnecessary reallocations by calling
vec.reserve(num_elements_to_push);
Though, if you anyhow insert from an array, the more idomatic way is to use insert
.
push_back
inserts a single element, hence in the worst case you may encounter multiple reallocations.
For the sake of the example, consider the case where the initial capacity is 2 and increases by a factor of 2 on each reallocation. Then
std::vector<int> vec = 1 ;
vec.push_back(2);
vec.push_back(3); // need to reallocate, capacity is 4
vec.push_back(4);
vec.push_back(5); // need to reallocate, capacity is 8
You can of course prevent unnecessary reallocations by calling
vec.reserve(num_elements_to_push);
Though, if you anyhow insert from an array, the more idomatic way is to use insert
.
answered Aug 16 at 8:44
formerlyknownas_463035818formerlyknownas_463035818
23.9k4 gold badges32 silver badges81 bronze badges
23.9k4 gold badges32 silver badges81 bronze badges
add a comment |
add a comment |
The major contributing factor is going to be the re-allocations. vector
has to make space for new elements.
Consider these 3 sinppets.
//pushback
std::vector<int> vec = 1;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
//insert
std::vector<int> vec = 1;
int arr[] = 2,3,4,5;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
//cosntruct
std::vector<int> vec = 1,2,3,4,5;
To confirm the reallocations coming into picture, after adding a vec.reserve(5)
in pushback and insert versions, we get the below results.
Interesting how adding reserve brings down the time for push_back and insert to similar levels.
– jacobi
Aug 16 at 9:27
1
Why would one want to addreserve()
to theinsert()
version? I don't get the same performance hit as you when doing it though: quick-bench.com/4ioxDEyzxMl37C7eHfNICKk0Tpc
– Ted Lyngmo
Aug 16 at 9:32
@TedLyngmo I did with clang. Don't know whyclang
's insert is slower.
– Gaurav Sehgal
Aug 16 at 10:57
1
Wow, yeah, that was a surprise! ... and usingclang++
+libc++(LLVM)
made it even worse: quick-bench.com/jfcVMGkhgFRI33-2PMPR74cM1G0
– Ted Lyngmo
Aug 16 at 11:44
add a comment |
The major contributing factor is going to be the re-allocations. vector
has to make space for new elements.
Consider these 3 sinppets.
//pushback
std::vector<int> vec = 1;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
//insert
std::vector<int> vec = 1;
int arr[] = 2,3,4,5;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
//cosntruct
std::vector<int> vec = 1,2,3,4,5;
To confirm the reallocations coming into picture, after adding a vec.reserve(5)
in pushback and insert versions, we get the below results.
Interesting how adding reserve brings down the time for push_back and insert to similar levels.
– jacobi
Aug 16 at 9:27
1
Why would one want to addreserve()
to theinsert()
version? I don't get the same performance hit as you when doing it though: quick-bench.com/4ioxDEyzxMl37C7eHfNICKk0Tpc
– Ted Lyngmo
Aug 16 at 9:32
@TedLyngmo I did with clang. Don't know whyclang
's insert is slower.
– Gaurav Sehgal
Aug 16 at 10:57
1
Wow, yeah, that was a surprise! ... and usingclang++
+libc++(LLVM)
made it even worse: quick-bench.com/jfcVMGkhgFRI33-2PMPR74cM1G0
– Ted Lyngmo
Aug 16 at 11:44
add a comment |
The major contributing factor is going to be the re-allocations. vector
has to make space for new elements.
Consider these 3 sinppets.
//pushback
std::vector<int> vec = 1;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
//insert
std::vector<int> vec = 1;
int arr[] = 2,3,4,5;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
//cosntruct
std::vector<int> vec = 1,2,3,4,5;
To confirm the reallocations coming into picture, after adding a vec.reserve(5)
in pushback and insert versions, we get the below results.
The major contributing factor is going to be the re-allocations. vector
has to make space for new elements.
Consider these 3 sinppets.
//pushback
std::vector<int> vec = 1;
vec.push_back(2);
vec.push_back(3);
vec.push_back(4);
vec.push_back(5);
//insert
std::vector<int> vec = 1;
int arr[] = 2,3,4,5;
vec.insert(std::end(vec), std::begin(arr), std::end(arr));
//cosntruct
std::vector<int> vec = 1,2,3,4,5;
To confirm the reallocations coming into picture, after adding a vec.reserve(5)
in pushback and insert versions, we get the below results.
answered Aug 16 at 8:57
Gaurav SehgalGaurav Sehgal
6,2121 gold badge11 silver badges28 bronze badges
6,2121 gold badge11 silver badges28 bronze badges
Interesting how adding reserve brings down the time for push_back and insert to similar levels.
– jacobi
Aug 16 at 9:27
1
Why would one want to addreserve()
to theinsert()
version? I don't get the same performance hit as you when doing it though: quick-bench.com/4ioxDEyzxMl37C7eHfNICKk0Tpc
– Ted Lyngmo
Aug 16 at 9:32
@TedLyngmo I did with clang. Don't know whyclang
's insert is slower.
– Gaurav Sehgal
Aug 16 at 10:57
1
Wow, yeah, that was a surprise! ... and usingclang++
+libc++(LLVM)
made it even worse: quick-bench.com/jfcVMGkhgFRI33-2PMPR74cM1G0
– Ted Lyngmo
Aug 16 at 11:44
add a comment |
Interesting how adding reserve brings down the time for push_back and insert to similar levels.
– jacobi
Aug 16 at 9:27
1
Why would one want to addreserve()
to theinsert()
version? I don't get the same performance hit as you when doing it though: quick-bench.com/4ioxDEyzxMl37C7eHfNICKk0Tpc
– Ted Lyngmo
Aug 16 at 9:32
@TedLyngmo I did with clang. Don't know whyclang
's insert is slower.
– Gaurav Sehgal
Aug 16 at 10:57
1
Wow, yeah, that was a surprise! ... and usingclang++
+libc++(LLVM)
made it even worse: quick-bench.com/jfcVMGkhgFRI33-2PMPR74cM1G0
– Ted Lyngmo
Aug 16 at 11:44
Interesting how adding reserve brings down the time for push_back and insert to similar levels.
– jacobi
Aug 16 at 9:27
Interesting how adding reserve brings down the time for push_back and insert to similar levels.
– jacobi
Aug 16 at 9:27
1
1
Why would one want to add
reserve()
to the insert()
version? I don't get the same performance hit as you when doing it though: quick-bench.com/4ioxDEyzxMl37C7eHfNICKk0Tpc– Ted Lyngmo
Aug 16 at 9:32
Why would one want to add
reserve()
to the insert()
version? I don't get the same performance hit as you when doing it though: quick-bench.com/4ioxDEyzxMl37C7eHfNICKk0Tpc– Ted Lyngmo
Aug 16 at 9:32
@TedLyngmo I did with clang. Don't know why
clang
's insert is slower.– Gaurav Sehgal
Aug 16 at 10:57
@TedLyngmo I did with clang. Don't know why
clang
's insert is slower.– Gaurav Sehgal
Aug 16 at 10:57
1
1
Wow, yeah, that was a surprise! ... and using
clang++
+ libc++(LLVM)
made it even worse: quick-bench.com/jfcVMGkhgFRI33-2PMPR74cM1G0– Ted Lyngmo
Aug 16 at 11:44
Wow, yeah, that was a surprise! ... and using
clang++
+ libc++(LLVM)
made it even worse: quick-bench.com/jfcVMGkhgFRI33-2PMPR74cM1G0– Ted Lyngmo
Aug 16 at 11:44
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f57521335%2finsert-or-push-back-to-end-of-a-stdvector%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZxeQQY6MWDk,v8ZVwQlRk6hTdBscnm,9cnR1FMjti8a IMpy5xgLcSTNOr8o5 1z,grtUrhvwfpgNRGupojF q,O1Yt7ePh,UcY
7
There is also another option:
std::vector<int> vec 1,2,3,4,5 ;
– JeJo
Aug 16 at 8:34
10
Create a small test program where you do each a million times. Build with optimizations enabled, and test and measure.
– Some programmer dude
Aug 16 at 8:34
1
Oh and while the end result (a vector with five elements) the two methods do different things. The most important difference is the allocation and reallocation of the data needed for the elements in the vector. The first variant could lead to four reallocations, and with a possibly different capacity and size, while the second variant there's probably only a single reallocation and maybe the same capacity and size.
– Some programmer dude
Aug 16 at 8:38
1
Personally I like method 2 because it's nice and concise and inserts all the new elements from an array in one go. That's the important reason, performance is secondary.
– john
Aug 16 at 8:43